Java集合体系
- Set(接口):代表无序、不可重复的集合
- List(接口):代表有序、重复的集合
- Map(接口):代表具有映射关系的集合
- Queue(接口):代表队列集合
集合类和数组区别
- 数组元素既可以是基本类型的值,也可以是对象(实际上保存的是对象的引用变量)。
- 集合里只能保存对象(实际上保存的是对象的引用变量,但通常习惯认为集合里保存的是对象)
Java的集合类主要由两个接口派生而出,他们是Java集合框架的根接口,这两个接口又包含了一些子接口或实现类。
UML关系图如下所示
PantUML语法
1 2
| A <|-- B //B继承A C <|... D //D实现了C
|
Collection
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118
| @startuml
interface Iterable{ }
interface Collection{ }
interface Set{ }
interface Queue{ }
interface List{ }
interface SortedSet{ }
class HashSet{ }
abstract AbstractSet{ }
abstract AbstractCollection{ }
class ArrayList{ }
abstract class AbstractList{ }
class Vector{ }
interface Deque{ }
class PriorityQueue{ }
abstract class AbstractQueue{ }
class TreeSet{ }
interface NavigableSet{ }
class LinkedHashSet{ }
class LinkedList{ }
abstract class AbstractSequentialList{ }
class Stack{ }
class ArrayDeque{ }
Iterable <|-- Collection Collection <|-- Set Collection <|-- Queue Collection <|-- List
Set <|-- SortedSet
AbstractSet <|-- HashSet Set <|.. HashSet
AbstractCollection <|-- AbstractSet Set <|.. AbstractSet
Collection <|.. AbstractCollection
AbstractList <|-- ArrayList List <|.. ArrayList
AbstractCollection <|-- AbstractList List <|.. AbstractList
AbstractList <|-- Vector List <|.. Vector
Queue <|-- Deque
AbstractQueue <|-- PriorityQueue
AbstractCollection <|-- AbstractQueue Queue <|.. AbstractQueue
AbstractSet <|-- TreeSet NavigableSet <|.. TreeSet
SortedSet <|-- NavigableSet
HashSet <|-- LinkedHashSet Set <|.. LinkedHashSet
AbstractSequentialList <|-- LinkedList List <|.. LinkedList
AbstractList <|-- AbstractSequentialList
Vector <|-- Stack
AbstractCollection <|-- ArrayDeque Deque <|.. ArrayDeque
@enduml
|
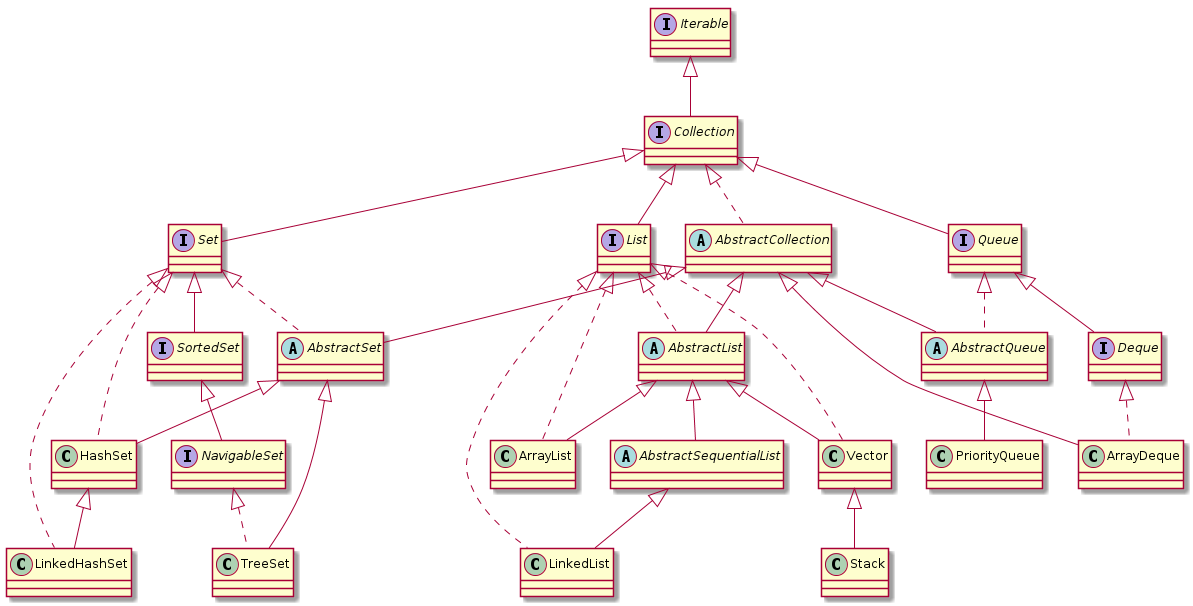
Map
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| @startuml
interface Map{ }
class HashMap{ }
abstract class AbstractMap{ }
class Hashtable{ }
abstract class Dictionary{ }
interface SortedMap{ }
class LinkedHashMap{ }
class Properties{ }
class TreeMap{ }
interface NavigableMap{ }
AbstractMap <|-- HashMap Map <|.. HashMap
Map <|.. AbstractMap
Dictionary <|-- Hashtable Map <|.. Hashtable
Map <|-- SortedMap
HashMap <|-- LinkedHashMap Map <|.. LinkedHashMap
Hashtable <|-- Properties
AbstractMap <|-- TreeMap NavigableMap <|.. TreeMap
SortedMap <|-- NavigableMap
@enduml
|
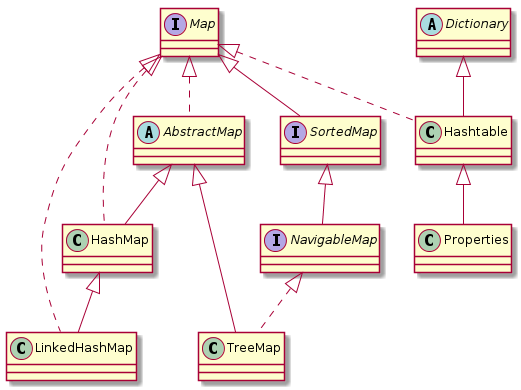
对于Set、List、Queue、Map四种集合,最常用的分别是:HashSet、TreeSet、ArrayList、LinkedList、ArrayDeque、HashMap、TreeMap。